Branching in JavaScript
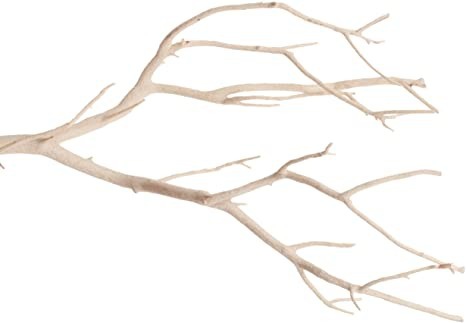
1. if-if else-else
The most common method to set part of your code under specific condition is to use if-else
, where if
part will be called if conditions are met and else
if not. Also we can use else if
combination, when we have several part of code that 100% sure shouldn't be called at the same time.
const a = true;
const b = true;
const c = false;
// Output: This log will be printed in console
if (a) {
console.log("This log will be printed in console");
}
// Output: This one will be printed instead
if (!b) {
console.log("This won't");
} else {
console.log("This one will be printed instead");
}
// Output: This one will be displayed
if (a && c) {
console.log("This one also will be ignored");
} else if (a && b) {
console.log("This one will be displayed");
}
// Btw, we can continue if-else if series as long as we want, and even add else for case, when no conditions met
2. Ternary (conditional) operator
This operator has the following form: condition ? ifTrue : ifFalse
, where first part is condition, pretty same as we used for if-else
, second - if condition met, and the third one - if not.
const a = true;
const b = false;
// Output: Thats true
console.log(a ? 'Thats true' : 'Thats false');
// Output: Thats false
console.log(b ? 'Thats true' : 'Thats false');
// You can also use nested ternary operator, but I not recomment to do so, because it will make your code less readable
// Output: Thats true in false
console.log(b ? 'Thats true' : a ? 'Thats true in false' : 'Just false');
3. && and || operators
This one is a bit more trickier.
Operator ||
will return first value that was considered as true
(non-empty string, not zero number, object, array, true
), or if there is no such value, it will return last false
value (false
, empty string, number 0
, null
, undefined
).
const a = null || 'its a';
const b = 'its b' || null;
const c = 'its c' || 'its d';
const d = undefined || null;
// Output: its a
console.log(a);
// Output: its b
console.log(b);
// Output: its c
console.log(c);
// Output: null
console.log(d);
Operator &&
will check values one by one and return the last value if all conditions met (or all values considered as true
), or first value that was considered as false
.
const a = null && 'its a';
const b = 'its b' && null;
const c = 'its c' && 'its d';
const d = undefined && null;
// Output: null
console.log(a);
// Output: null
console.log(b);
// Output: its d
console.log(c);
// Output: undefined
console.log(d);
4. Switch statement
This one uses expression that was set in switch
part, and evaluate it with statements placed in case
. If it doesn't match any statement, default
part called.
const tea = 'green';
switch(tea) {
case 'black':
console.log('black tea');
break; // need to break each time to prevent calling another blocks of code that doesn't even match
case 'green':
console.log('green tea');
break;
case 'oolong':
console.log('oolong tea');
break;
default:
console.log('no such tea');
break;
};